Cython tutorial: How to speed up Python
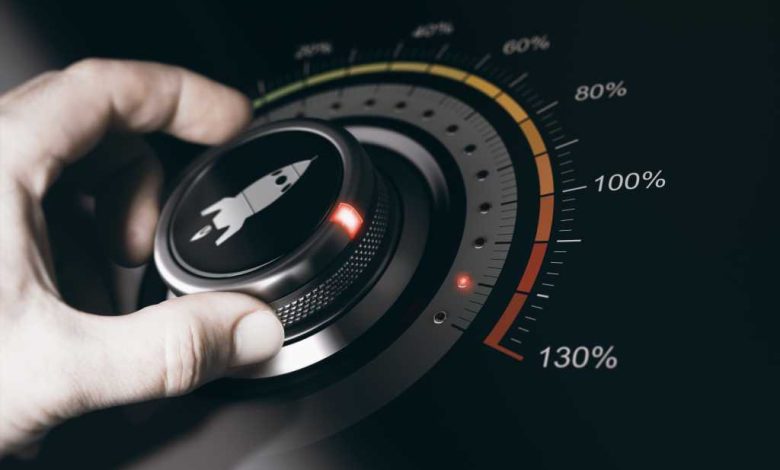
Python’s profiler, cProfile
, generates stories that present which features take up probably the most time in a given Python program. By default, Cython code doesn’t present up in these stories. Nonetheless, you possibly can allow profiling on Cython code by inserting a compiler directive on the high of the .pyx
file with features you wish to embody within the profiling:
# cython: profile=True
You can too allow line-by-line tracing on the C code generated by Cython. Doing this imposes loads of overhead, so it’s turned off by default. (Profiling usually imposes overhead, so be sure you toggle it off for code that’s being shipped into manufacturing.)
Cython may also generate code stories that point out how a lot of a given .pyx
file is being transformed to C, and the way a lot of it stays Python code. The setup.py
file from our instance specified this with the annotate=True
assertion within the cythonize()
perform.
Delete the .c
recordsdata generated within the challenge and re-run the setup.py
script to recompile every thing. Once you’re achieved, you need to see an HTML file in the identical listing that shares the title of your .pyx
file—on this case, num.html
. Open the HTML file and also you’ll see the elements of your code which might be nonetheless depending on Python highlighted in yellow. You may click on on the yellow areas to see the underlying C code generated by Cython.
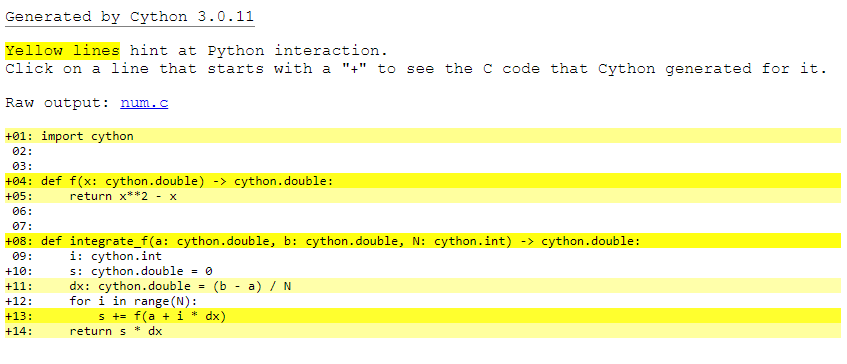
A Cython code annotation report. The yellow highlights point out elements of the code that also rely on the Python runtime.
IDG
On this case, the def f
perform remains to be highlighted in yellow, regardless of having its variables explicitly typed. Why? As a result of it’s not annotated explicitly as a Cython perform. Cython assumes any perform not annotated with @cython.cfunc
is only a Python perform, and never convertible to pure C.
You may repair this by modifying the def f
declaration to learn:
@cython.cfunc
def f(x: cython.double) -> cython.double:
Save and recompile the file, and reload the report. You need to now see the def f
perform is not highlighted in yellow; it’s pure C.
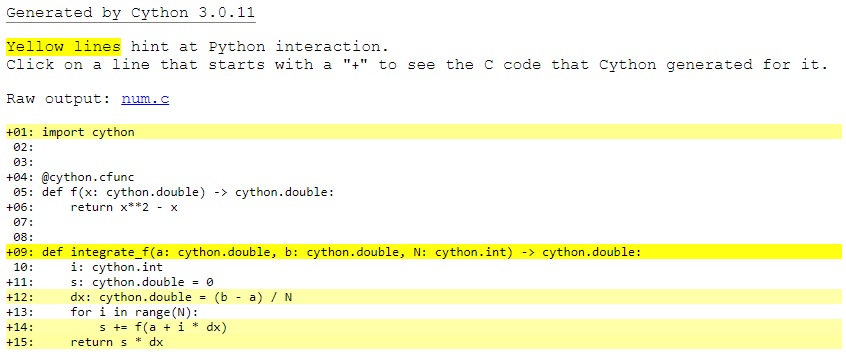
The revised Cython perform, now pure C, generates no highlights.
IDG
Observe that when you’ve got profiling enabled as described above, even “pure” C features will present some highlighting, as a result of they’ve been adorned with hint code that calls again into Python’s internals.
Additionally observe that the division operation in line 12 can be highlighted; it’s because Cython routinely inserts assessments for division by zero, and raises a Python exception if that’s the case. You may disable this with the cdivision
compiler directive, or by the use of a decorator on the perform (@cython.cdivision(True)
).
Cython sources
Now that you’ve some thought of how Cython integrates with an current Python app, the subsequent step is to place it to work in your personal purposes, and to utilize different sources on the market: